Powershell Module for transferring files via SFTP
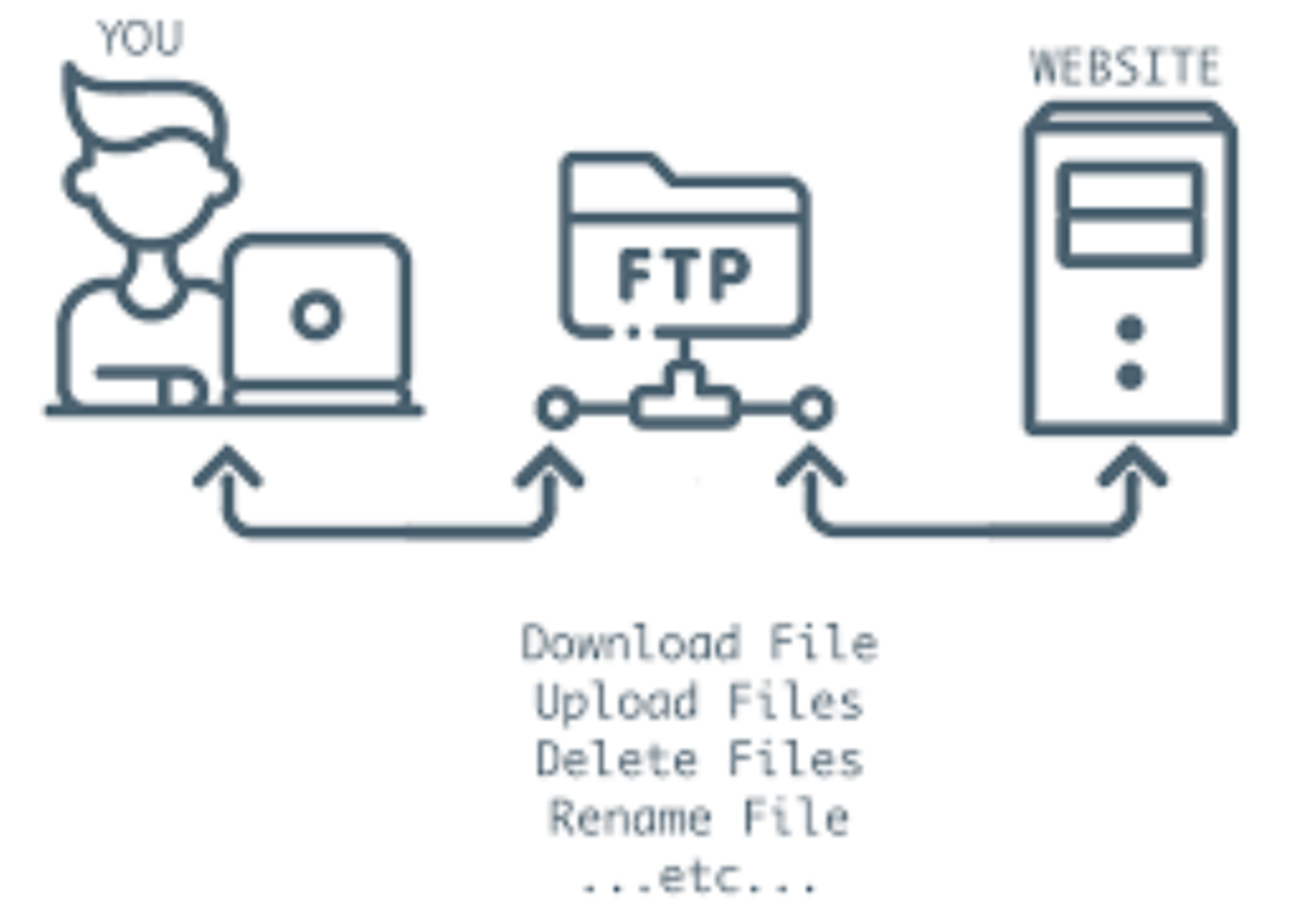
I had the need for several automated jobs to be able to exchange files securely with partners via the Secure FTP (SFTP). I chose powershell because the jobs:
- get executed on windows nodes. It is less overhead than creating console apps in .NET
- required things like querying databases, transforming data, and archiving files
I spent a little bit of time looking into different options, and decided to utilize the latest version of the WinSCP library provided the required options - such as designating the transfer mode, deleting source files, and specifying file masks.
I created a generic powershell module that exposes upload and download functions. The module is available on github:
https://github.com/jaygrossman/SftpPowershellModule
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
Function SFTPDownloadFiles {
Param(
[string] $Username, # Required. Username of SFTP account
[string] $Password, # Required. Password of SFTP account
[string] $HostName, # Required. HostName of SFTP server
[string] $RemotePath, # Required. Starting path of SFTP server
[string] $LocalPath, # Required. Starting path of local server
[string] $SshHostKeyFingerprint, # Required. Host Key,
ssh-rsa 1024 xx:xx:xx:xx:xx:xx:xx:xx:xx:xx:xx:xx:xx:xx:xx:xx
[string] $FileMask, # Optional. Filter string to limit files to download
[bool] $Remove=$false, # Optional. Whether to remove original file
[string] $TransferMode="binary"# Optional. Transfer can be binary, ascii, or automatic
)
Function SFTPUploadFiles {
Param(
[string] $Username, # Required. Username of SFTP account
[string] $Password, # Required. Password of SFTP account
[string] $HostName, # Required. HostName of SFTP server
[string] $RemotePath, # Required. Starting path of SFTP server
[string] $LocalPath, # Required. Starting path of local server
[string] $SshHostKeyFingerprint, # Required. Host Key,
ssh-rsa 1024 xx:xx:xx:xx:xx:xx:xx:xx:xx:xx:xx:xx:xx:xx:xx:xx
[string] $FileMask, # Optional. Filter string to limit files to upload
[bool] $Remove=$false, # Optional. Whether to remove original file
[string] $TransferMode="binary"# Optional. Transfer can be binary, ascii, or automatic
)
}